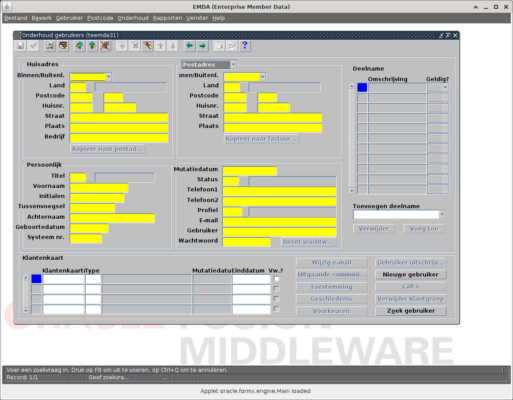
Oracle Forms Standalone Runner
TL;DR; See https://github.com/atkaper/oracle-forms-standalone-runner on how to download and run the forms viewer… If you want the background as to how I got there, read on…
At the office, we still use a couple of old/legacy oracle-forms-11 applications. The “normal” (designated) users will run these from Windows, in the Edge browser switched to old Internet-Explorer compatibility modeβ¦ You can not start Oracle Forms Applets in any other browser or operating system, as applets are no longer supported.
As I do run a Linux machine, I do not have Edge, and needed a different way to run the Oracle-Forms applications. To fix this issue, I went looking around a bit on the internet. A java applet can be started outside of a browser, so it was just a matter of finding out how.
Java 8 has a command “appletviewer” which can be used to start an applet from the command line. This however requires you to have the applet source-code, and in there you need to add annotations to get it started properly. This was no option for me (see for example: https://www.geeksforgeeks.org/different-ways-to-run-applet-in-java/).
I did find a couple of java code examples on the internet, which can start an applet. From those, I started testing using this small example (from https://ecomputernotes.com/java/awt-and-applets/appletfromcommandline):
import java.applet.Applet;
import java.awt.*;
import java.awt.event.*;
public class CommandLineApplet extends Applet {
public static void main(String[] args) {
Frame comApplet = new Frame("Applet from Command Line");
comApplet.setSize(350, 250);
Applet commandLineApplet = new CommandLineApplet();
comApplet.add(commandLineApplet);
comApplet.setVisible(true);
comApplet.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
public void paint(Graphics g) {
g.setFont(new Font("Arial",Font.BOLD,14));
g.drawString("Applet from Command Line", 100, 100);
}
}
Code language: Java (java)
In this example, I added the jar files I could find on the Oracle Forms start URL/HTML page, and changed the line which instantiates the applet:
// Old line:
Applet commandLineApplet = new CommandLineApplet();
// New lines:
Class classType = Class.forName("oracle.forms.engine.Main");
Applet commandLineApplet = (Applet) classType.newInstance();
// Later added lines:
commandLineApplet.init();
commandLineApplet.start();
Code language: Java (java)
No luck… The two bottom lines actually give a clue as to what goes wrong, as in that case I got an exception:
Exception in thread "main" java.lang.NullPointerException
at java.applet.Applet.getParameter(Applet.java:191)
Code language: CSS (css)
Conclusion, the simple example above is not good enough π Back to searching the next example(s), and I concentrated in the search on finding code which could pass on the applet parameters we need…
The best example I found was this one:
http://www.java2s.com/Code/Java/Swing-JFC/AppletViewerasimpleAppletViewerprogram.htm, created by Ian Darwin. Next to starting the applet, it has a partial implementation of AppletContext and AppletStub interface functions, which can be used by the Applet to talk to its startup parent. These context and stub methods also had the one to read the applet parameters, this is what I needed.
To complete the example to a usable solution, a number of changes and additions were needed:
- Read a given URLs HTML document, to gather the applet start parameters from (e.g. read the start URL as used by the Windows/Edge users).
- Pitfall/Initial-Issue: the values in the parameters needed a HTML-decode. Took me a while to see that, as the exception I got from the applet was really really cryptic π
- Two of the parameter values I had to prefix with the base-URL of the page from which the start HTML was read. This was needed to simulate as if the applet was started from a browser.
- Implemented property override function to be able to use different settings locally, as opposed to what was in the web start HTML page. I use this to let Oracle Forms know that I want to stay in the parent window, and not start a second screen.
- Implement and update the callback methods of the Applet-Context/Stub as much as possible.
- Important one: the parameter reader.
- Image retrieval.
- URL in browser open function.
- Code/Document-base functions.
- And more…
- Added workarounds/fixes to get the program to terminate properly when you closed all Oracle Forms windows in the applet. I ended up counting the number of displayed components, and if none are found, then exit.
- Add code to dynamically load the needed applet JAR files in memory (as indicated in the the HTML document), and add them to the class-path. I started my test by manually downloading the JARs and adding them to the command line class-path on the java start command. But I wanted all to be automatic. No manual steps, and no extra download scripts are needed this way.
- Refactor the code, and split the single source in three classes.
- Added an Oracle Forms override class to fix issues with my font size being too big. You can now configure screen scaling, and font size to use. See readme on GitHub.
- β see status update at end of document; I also needed to add cookie handling.
The result of all of this can be found in the GitHub project: https://github.com/atkaper/oracle-forms-standalone-runner
Instructions for use/start are simple:
- make sure you have a working java 8 runtime installed (newer ones do not have applet functions in them).
- download my compiled executable (jar) once: https://github.com/atkaper/oracle-forms-standalone-runner/raw/master/target/oracle-forms-runner-1.0.0-SNAPSHOT.jar (if you want, you can build it yourself from the source-code in the git repository – but no need, the jar works fine).
- start it using:
java -Doverride_separateFrame=false -jar oracle-forms-runner-1.0.0-SNAPSHOT.jar "http://eforms-tst3.ecom.somewhere.nl:8888/forms/frmservlet?config=emda"
in above start, make sure that either the java 8 context is default (by setting PATH and JAVA_HOME), or just prefix the java command by the exact location where your java 8 executable is stored. On my machine for example I use: ~/bin/java/jdk8/bin/java
instead of just java
as I have multiple versions of java installed.
Demo screenshots:
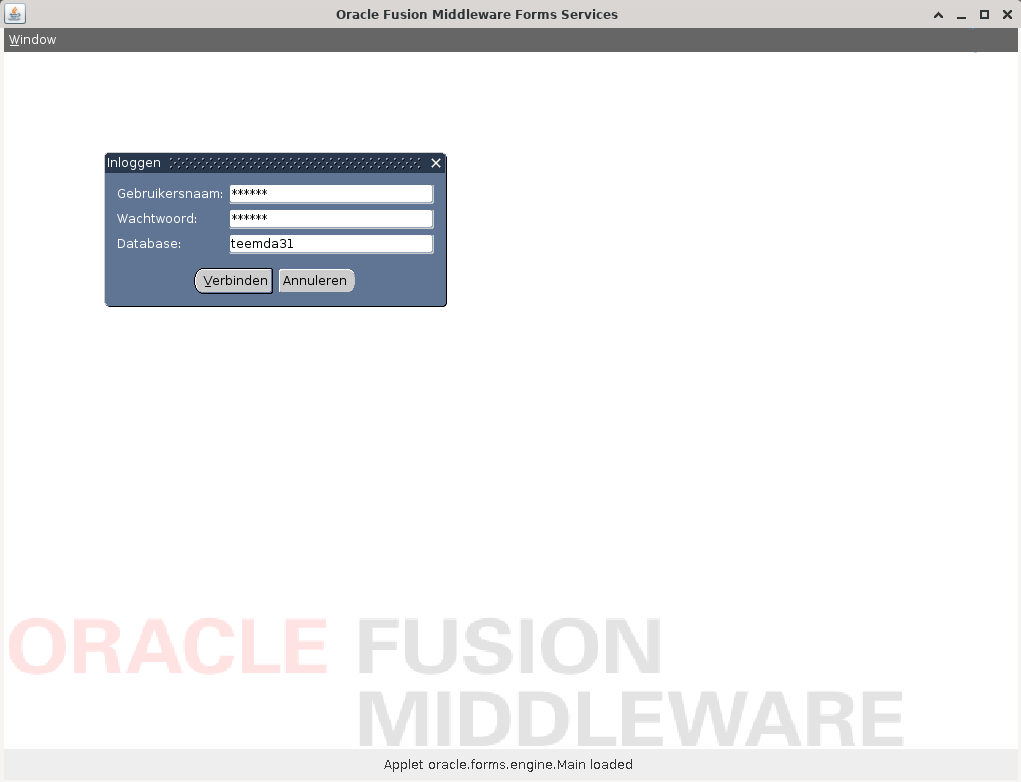
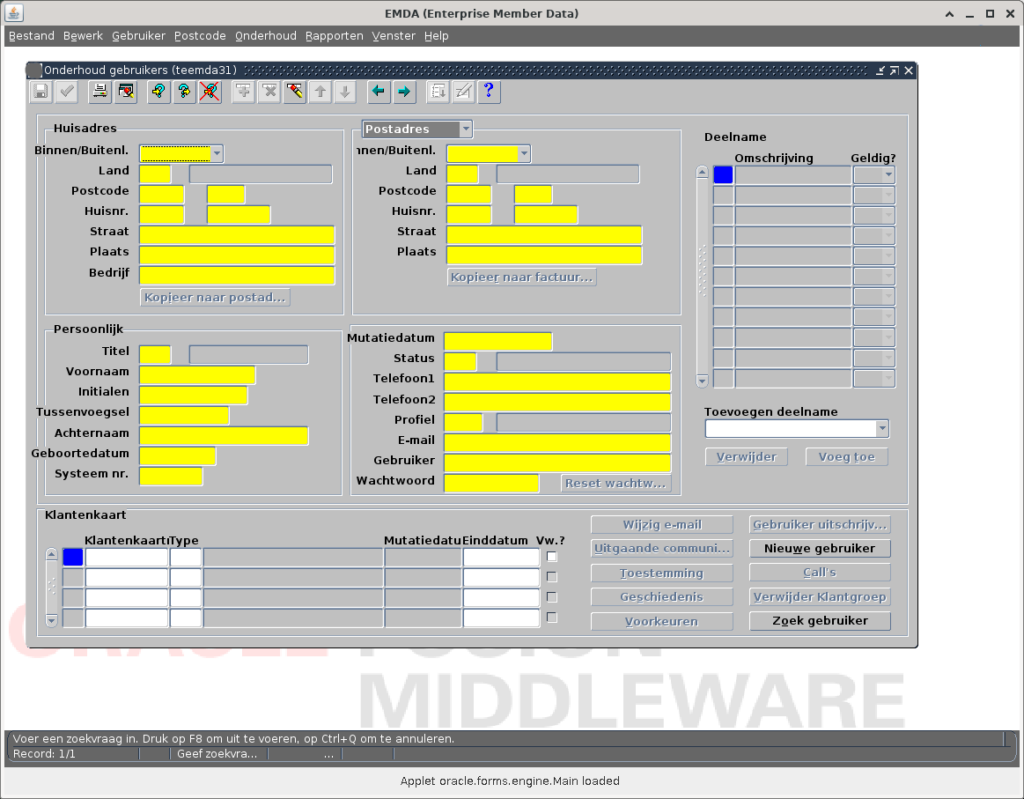
Note: I have made a new version, which supports scaling the screen, and font-size. Here’s a partial screenshot of the fixed version:
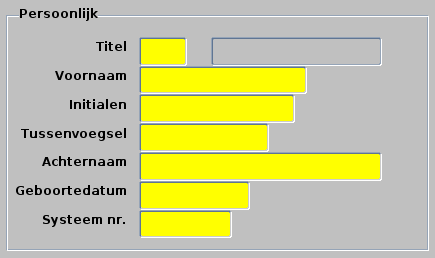
# example start, for high-resultion monitor, scaling down the font, and using a custom background:
java \
-Doverride_background="https://www.kaper.com/wp-content/uploads/2022/10/blue-backdrop.jpg" \
-Doverride_webformsTitle="Emda T3" \
-Doverride_separateFrame=false \
-Doverride_mapFonts=yes \
-Doverride_clientDPI=120 \
-Doverride_downsizeFontPixels=3 \
-jar oracle-forms-standalone-runner/target/oracle-forms-runner-1.0.0-SNAPSHOT.jar \
"http://eforms-tst3.ecom.somewhere.nl:8888/forms/frmservlet?config=emda"
Code language: PHP (php)
Conclusion:
I do not have much experience with building Applets, but it was quite nice that I did get this to work! It’s great that I do not have to find a workaround to run Edge, or use a remote desktop.
At the moment, no one else has tried using this Oracle-Forms Launcher, and I am really curious to know if it does work for anyone else! And if it does work on Mac-OS. But I think it should be fine. β It does work on MacOS, as long as you use the proper JDK (see bottom of the readme in the github project).
My use-case was starting Oracle Forms, but I think you should be able to use this code, or just add some small tweaks to it, to start other (old) applets as well. Just make sure to look at the HTML which starts the applet, and possibly update the source to read the parameters from it if my version does not pick up the right ones.
Note: during my quest of finding a way to start applets, I also came across a Chrome browser plugin (CheerpJ Applet Runner) which runs applets using JavaScript to simulate java 8. This did do some startup for Oracle Forms, but gave me the same exception message I got when I did not yet HTML decode the start parameters! Perhaps that applet launcher plugin also would work, if we just edited the HTML start page π I did not try that.
Thijs, September 24, 2022.
Status update:
I did notice last week that the Launcher did not work on our Load-Balanced PRODUCTION servers… The Oracle Forms Applet does not read and pass back the cookies from the servers properly. It did depend on running in a browser to let the browser handle those. It would give me this error:
"FRM-93618: Fatale fout bij lezen gegevens van runtimeproces. Neem contact op met de systeembeheerder."
"FRM-93618: fatal error reading data from runtime process. Contact your system administrator."
Code language: JSON / JSON with Comments (json)
A fix / workaround was needed π And of course this fix has been build!
I have altered the Launcher code to add a special Cookie-Retaining-Proxy between the Forms Applet, and the back-end servers. This is needed to keep sticky sessions with the proper load-balanced back-end server instance. Just download the latest version of the executable from the GitHub project to make use of this. The README in GitHub has some more details on that part.
I also found a nice bling-bling override parameter, with which you can choose your own background image.
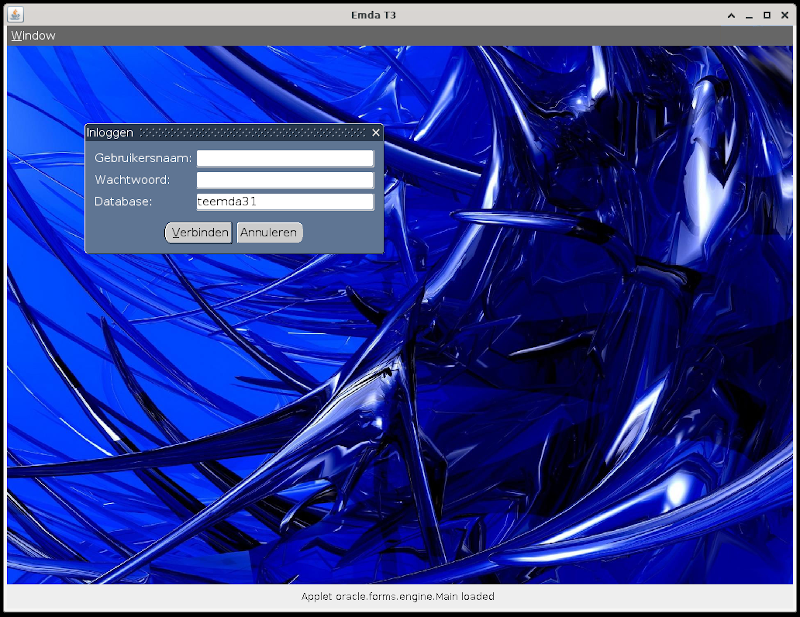
Just add the next parameter to your command line:
-Doverride_background="https://www.kaper.com/wp-content/uploads/2022/10/blue-backdrop.jpg"
Code language: JavaScript (javascript)
Thijs, October 7, 2022.
Update:
As feature request from Bogdan, I have added the option to override the Registry.dat server file, by tweaking the embedded proxy code. You can now specify one or more url path’s to return local files.
See https://github.com/atkaper/oracle-forms-standalone-runner#override-server-files for more information.
It boils down to the following; download your original server Registry.dat file, on server location/path: /forms/registry/oracle/forms/registry/Registry.dat, and put it in a local file. Tweak the contents as needed, and then add a start option similar to this:
-Dlocalfile_reg=".*Registry.dat:/yourfolder/MyRegistry.dat:text/plain"
Code language: JavaScript (javascript)
For Oracle 11, you can not override color scheme’s using this, but for Oracle 12 that should be possible.
I might have a look later at different ways to change color scheme’s, to get that working for Oracle 11 apps as well.
Thijs, July 2, 2023.
Note: do not forget to rebuild from source, or download the latest copy of the JAR: https://github.com/atkaper/oracle-forms-standalone-runner/raw/master/target/oracle-forms-runner-1.0.0-SNAPSHOT.jar
(it is still using the same version in the jar filename, sorry, was too lazy to update on each build).
4 thoughts on “Oracle Forms Standalone Runner”
Very nice, awesome job!
It would be awesome if we would have the ability to change the font that is being used (in case you’re just the end user and you can’t do that from the server), and maybe even the colors!
Colors may be a bit too much already, but at least the font would be amazing.
Thanks again!
Hi MRW, I have added the option to override the Registry.dat file from the server by a local file. See https://github.com/atkaper/oracle-forms-standalone-runner/tree/master#override-server-files for more information (or the bottom of the blog post). You can override the fonts in there, and for Oracle 12 (not 11), you can add custom color scheme’s in there as well (at least, I’m told so. Could not test it myself).
Nice work, but did you know there is a tool from oracle that already does what you have done?
https://docs.oracle.com/en/middleware/developer-tools/forms/12.2.1.19/working-forms/forms-standalone-launcher.html#GUID-9581A327-D239-4F5C-925C-169E60B5F379
This tool is for forms12, but migrating from 11g to 12c is not a hard step. Basically you need to reinstall the forms server and compile the forms again.
on the other side, althoug is not certifijavaweb
Yes I know π Your sentence “Basically you need to reinstall the forms server and compile the forms again” is the exact reason why I create my runner. I have no control over the server in any way. Only access from outside to it. But thanks for the heads-up anyway!